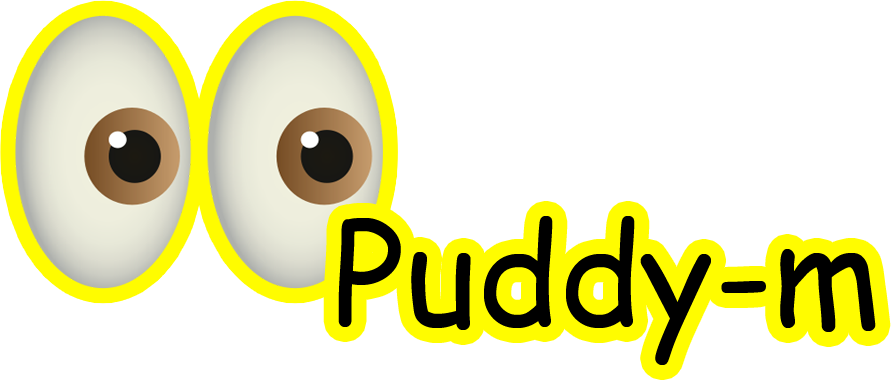
Make use of a promise based library to make quick validations on your code!
Install using the NPM Package Manager
>$
npm install puddy-m
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
import { validateObjectWithKeys } from "puddy-m/lib/validators/complexValidators";
import { validateString, validateNumber } from "puddy-m/lib/validators";
const express = require('express')
const app = express()
app.get('/', function (req, res) {
const { body } = req
validateObjectWithKeys(headers, null, "username", "age")
.then(req => validateString(req["username"], []))
.then(tuple => validateNumber(req["password"], tuple))
.then(([username, password])=>{
// Handle valid logic.
})
.catch(e=>{
//Handle the rejection logic
res.send("The parameter username or age weren't sent in the request or they got invalid data type."))
}
})
app.listen(3000)
Use chained promise calls to help you efficiently validate variables, for example, handling express request object.
Versions